Authentication methods
There are three authentication methods to use the API:
- Simple browser authentication – for exports, datasheets, image links, quick viewing in the browser etc.
- ITscope Authentication – requires an active browser session on https:/www.itscope.com and should not be used programmatically. This method can e.g. be used for deep links from authorised participants to the platform.
- Programmatic Authentication via HTTP Basic Authentication – this is the standard authentication with username:password for the use of the ITscope API.
There are 2 different variants:- Personal API access for individual use of the API. This variant is described in the section further down.
- Interface to ITscope for partner systems, which implement a connection for shared customers. Our interface partners receive the respective instructions during the implementation on request.
Personal ITscope API credentials
Personal login information for the ITscope API can be viewed in the own employee profile. These are required for Username:Password HTTP Basic Authentication for the ITscope API.
Following data is required:
- Account ID – this is the username
- API Key – this is the password
This allows the ITscope API to be accessed.
If you need a new API key, you can generate one with the New API Key button. Caution: this will invalidate the old key!
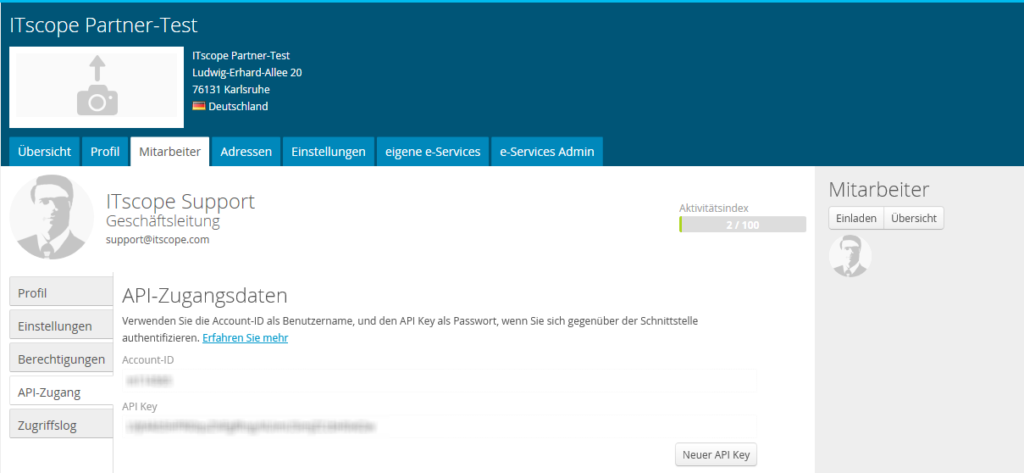
Programmatic Authentication via HTTP Basic Authentication
For programmatic authentication in the development code, the API user (account ID) and the API password (API key) must be specified in the header, encoded as Base64. To do this, you can use a service like https://www.base64encode.org and encode the Username:Password ‘<Account-ID>:<API Key>’ string. The result of the encoding must then be inserted into the HTTP header Authorization:Basic. If the API URL is entered interactively into the browser, authentication is performed using the browser’s standard mechanisms for ‘Basic Auth’.
Example pseudo code for an API request:
Example pseudo code for a call via the API ITscope including mandatory HTTP header user-agent:
... // api url without credentials, e.g. string apiurl = "https://api.itscope.com/2.1/products/search/ean=0888462025737/standard.xml"; HttpWebRequest request = (HttpWebRequest)WebRequest.Create(apiurl); // username:password Combination Base64 encoded string credentials = <Account-ID>+ ":" + <API Key> ; authorization = Convert.ToBase64String(Encoding.Default.GetBytes(credentials)); // Basic Authorization as http header request.Headers["Authorization"] = "Basic " + authorization; // useragent in the form <applicationCompany> - <applicationName> - <applicationVersion> // eg my company-my application-4.0927 request.UserAgent = "MyCompany-My-Application-4.0927" //Others request.Method = "GET" request.KeepAlive = True request.ContentType = "application/xml" request.AllowAutoRedirect = true ...
Examples of programmatic authentication
Example pseudo code for using a generated C # client directly from the Swagger Framework of the ITscope API including mandatory HTTP header user-agent
...
Configuration config = new Configuration();
// username:password combination Base64 encodedstring credentials= ApiClient.Base64Encode("<Account-ID>:<API Key>");
// Basic Authorization as http headerconfig.AddDefaultHeader("Authorization", "Basic" +
credentials);
// useragent in the form <applicationCompany> - <applicationName> - <applicationVersion> // eg my company-my application-4.0927config.setUserAgent("MyCompany-MyApplication-4.0927"); // misc ProductsApi pApi = new ProductsApi(config);
...
Example pseudo code header for a PHP script:
....
// username:password combination Base64 encoded // Basic Authorization as http header header('Authorization: Basic '.base64_encode("
<Account ID>:<API Key>"));
// useragent in the form <applicationCompany> - <applicationName> - <applicationVersion> // e.g. my company-my application-4.0927 header('UserAgent:MyCompany-MyApplication-4.0927');....
Example pseudo code header for a PHP script with curl:
// username:password combination not Base64 encoded when the curl option CURLAUTH_BASIC is set, // then curl executes a Base64 encoding itself $UserPWD = "<Account ID>:<API Key>"; $url = "https://api.itscope.com/2.1/products/search/ean=
0888462025737/standard.xml"; $UserAgent = $_SERVER['HTTP_USER_AGENT']; $pptions = array (CURLOPT_URL => $url, CURLOPT_HEADER => true, CURLOPT_USERAGENT => $UserAgent, CURLOPT_HTTPAUTH => CURLAUTH_BASIC, CURLOPT_USERPWD => $UserPWD); $ch = curl_init(); curl_setopt_array($ch, $options); $Data = curl_exec($ch); ....
Simple browser authentication
The easiest way to call up the export via browser, wget or in Windows task planning etc. is to pass the authentication directly in the URL (the user must always have an entry in the Account ID field, see above!)
Note: this method does not work in Internet Explorer.
An example of a retrieval in the browser for an export
https://<Account-Id>:<API Key>@api.itscope.com/2.1/products/exports/<EXPORT-ID>
If you do not enter the user name <accountID> or the password <API key> when retrieving the URL, authentication is performed via the standard mechanism of the browser for ‘Basic Auth’ and you are prompted to enter the credentials in a separate window.
ITscope authentication
This type of authentication is primarily intended for deeplinks into the platform and requires an active session on ITscope. If no active session exists, you will be directed to the login page. To do this, append the API URL with ‘itscope’ for the ‘auth’ query parameter. A call then looks as follows:
https://api.itscope.com/2.1/products/ean/0886111583935/deeplink.html?auth=itscope